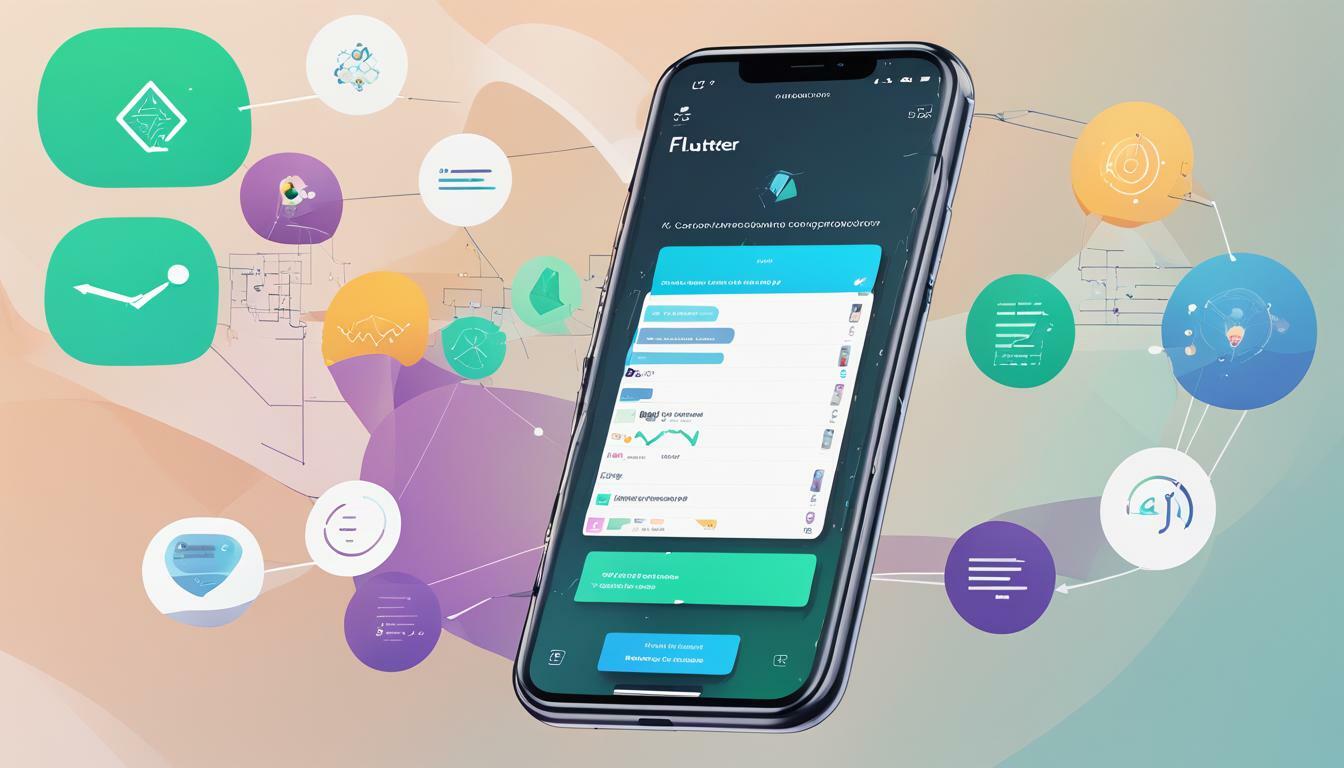
Welcome to our comprehensive guide on elevating your Flutter projects through the use of Dart programming. In today’s world where mobile app development has become a necessity, cross-platform app development has gained popularity, and Flutter is one such framework that is widely used. However, to create high-quality Flutter apps, it’s essential to have a strong foundation in Dart programming language. That’s why this article focuses on providing you with comprehensive Dart development guidelines that will help improve your Flutter coding skills.
Understanding Flutter Projects and Dart Development
Flutter has quickly become one of the most popular frameworks for mobile app development. It allows developers to create high-performance, visually appealing, and cross-platform mobile apps. Dart programming language is the backbone of Flutter, providing a fast and efficient way to develop apps for both iOS and Android platforms.
When developing Flutter apps, it’s essential to understand the basics of Flutter projects and Dart development. A Flutter project consists of both Dart code and a set of files that define the app’s structure, style, and behavior. Dart programming language is an object-oriented language that allows developers to write concise and readable code. It has many features that make it easy to use, such as optional typing, garbage collection, and asynchronous programming support.
Flutter app development involves using Dart programming language to write code to build UI components, handle user input, manage app state, and interact with backend services. Dart comes with a rich set of libraries and frameworks that help developers create complex apps quickly. Flutter also provides many built-in widgets that make it easy to create beautiful and responsive UI designs.
Developers can use the Dart programming language to build both native and web apps. Flutter provides the necessary tools and libraries to compile Dart code to native ARM code, which runs directly on the device. This ability to write once and deploy everywhere is what makes Flutter so popular for cross-platform mobile app development.
Comprehensive Dart Development Guidelines
Developing Flutter projects with Dart programming requires a comprehensive set of guidelines to ensure optimal performance and maintainability. Below are some best practices and coding conventions to follow:
Guideline | Description |
---|---|
Always use the latest version of Dart and Flutter | Update your development environment regularly to ensure you’re using the latest stable versions of Dart and Flutter. |
Adhere to the Dart style guide | Consistently apply the Dart style guide to improve readability and maintainability of your code. Use tools such as Dartfmt to automate formatting. |
Use packages and plugins from trusted sources | Make sure to use packages and plugins from trusted sources to ensure compatibility with new versions of Flutter and prevent security vulnerabilities. |
Minimize the use of external dependencies | Use external dependencies sparingly to avoid unnecessary code bloat and potential security risks. Whenever possible, use built-in Flutter widgets and libraries instead of third-party packages. |
These guidelines, when followed consistently, can help improve your Dart development and maintainable codebase. Always strive for simplicity and readability in your Flutter coding, while keeping performance and scalability in mind.
Enhancing Flutter Projects with Practical Coding Examples
In this section, we’ll explore various practical coding examples that demonstrate how to enhance Flutter projects using Dart programming. These examples cover UI design, state management, navigation, and integration with backend services. By following these coding examples, you’ll be able to optimize your Flutter app development and ensure efficient and effective mobile app development.
UI Design
The user interface (UI) is an essential aspect of any mobile app development. Creating a visually appealing and user-friendly UI can significantly improve the user experience. One way to enhance your Flutter projects’ UI design is by using the Flutter Widgets .
Widget | Description |
---|---|
Container | A rectangular box that can contain other widgets |
Text | A widget that displays a string of text |
Icon | A widget that displays an icon |
With these Flutter Widgets, you can create custom UI designs. For instance, you can use the Container
widget to create a custom button and use the Text
widget to add text to the button.
State Management
Effective state management is crucial for any Flutter project. State management refers to the management of data and UI state that changes over time. One approach to state management in Flutter is using the Provider package .
The Provider package enables you to create a central repository for data and state. It also allows you to access and modify the data and state across different widgets with ease.
Navigation
Navigation is another critical aspect of any mobile app development. Flutter provides support for both Material Design and Cupertino navigation patterns. You can use the Navigator
class to implement navigation between different pages or screens in your Flutter project.
Here’s an example of how to use the Navigator
class to navigate between screens:
Navigator.push(context, MaterialPageRoute(builder: (context) => Screen2()));
In this example, calling the Navigator.push()
method with the target screen’s builder function as a parameter will navigate to the target screen.
Integration with Backend Services
Integration with backend services is essential for most mobile app development projects. Flutter provides support for various HTTP clients , making it easy to integrate with backend services.
Here’s an example of how to use the http package to make an HTTP request:
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/todos/1'));
In this example, the http.get()
method makes an HTTP GET request to the specified URL.
With these practical coding examples, you can enhance your Flutter projects and ensure efficient and effective mobile app development.
Conclusion
In conclusion, comprehensive Dart development guidelines are essential for elevating Flutter projects. By following best practices and applying efficient coding techniques, developers can enhance the performance and maintainability of their mobile apps. With an understanding of Flutter projects and Dart development fundamentals, developers can create cross-platform apps that meet the needs of their users.
It is important to continually explore resources and stay up to date with new developments in the field. By doing so, developers can continue to improve their skills and create innovative mobile apps that meet the changing needs of users. With these comprehensive Dart development guidelines, developers can take their Flutter projects to the next level and create exceptional mobile apps.